Python Slots Speed
When running a complex Python program that takes quite a long time to execute, you might want to improve its execution time. But how?
First of all, you need the tools to detect the bottlenecks of your code, i.e. which parts take longer to execute. This way, you can concentrate in speeding these parts first.
Its CPU speed runs at 120MHz (Boost up to 200MHz). Realtek RTL8720DN chip supports both Bluetooth and Wi-Fi providing the backbone for IoT projects. The Wio Terminal itself is equipped with a 2.4” LCD Screen, onboard IMU(LIS3DHTR), microphone, buzzer, microSD card slot, light sensor, and infrared emitter(IR 940nm). Use slots when defining a Python class. You can tell Python not to use a dynamic dict, and only allocate space for a fixed set of attributes, eliminating the overhead of using one dict for every object by setting slots on the class to a fixed list of attribute names. Slots also prevent arbitrary attribute assignment on an object, thus the. Created on 2017-12-16 12:21 by pitrou, last changed 2018-01-15 18:34 by pitrou.This issue is now closed.
And also, you should also control the memory and CPU usage, as it can point you towards new portions of code that could be improved.
Therefore, in this post I’ll comment on 7 different Python tools that give you some insight about the execution time of your functions and the Memory and CPU usage.
1. Use a decorator to time your functions
The simpler way to time a function is to define a decorator that measures the elapsed time in running the function, and prints the result:
2 4 6 8 10 12 14 | from functools import wraps @wraps(function) t0=time.time() t1=time.time() (function.func_name,str(t1-t0)) returnresult |
Then, you have to add this decorator before the function you want to measure, like
2 | def myfunction(...): |
For example, let’s measure how long it takes to sort an array of 2000000 random numbers:
2 4 6 | def random_sort(n): if__name__'__main__': |
If you run your script, you should see something like
Total time running random_sort:1.41124916077seconds |
2. Using the timeit module
Anther option is to use the timeit module, which gives you an average time measure.

To run it, execute the following command in your terminal:
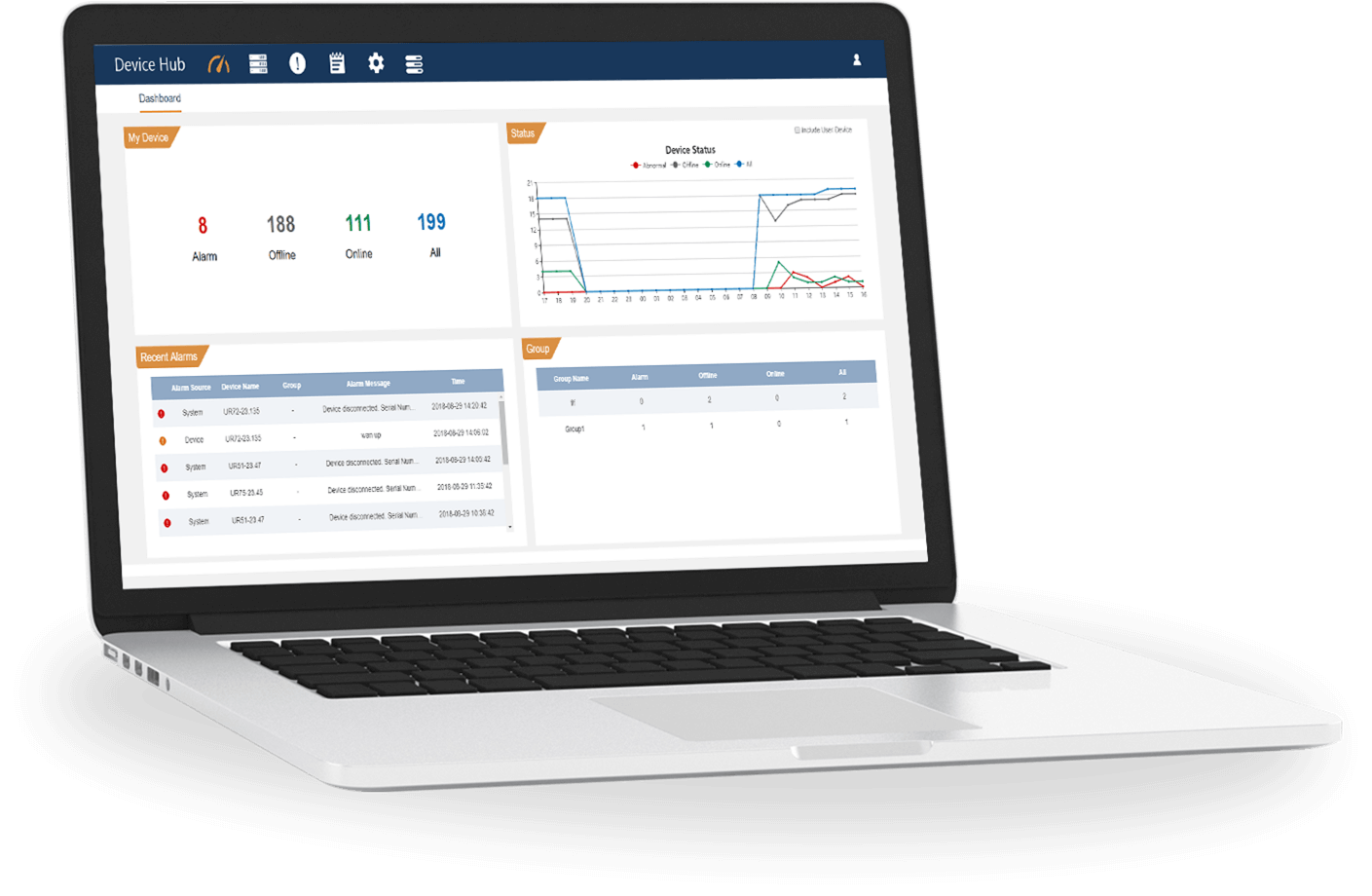
$python-mtimeit-n4-r5-s'import timing_functions''timing_functions.random_sort(2000000)' |
where timing_functions is the name of your script.
At the end of the output, you should see something like:
indicating that of 4 times running the test (-n 4), and averaging 5 repetitions on each test (-r 5), the best test result was of 2.08 seconds.
If you don’t specify the number of tests or repetitions, it defaults to 10 loops and 5 repetitions.
3. Using the time Unix command
However, both the decorator and the timeit module are based on Python. This is why the unix time utility may be useful, as it is an external Python measure.
To run the time utility type:
which gives the output:
The line_profiler module gives you information about the CPU time spent on each line in your code.
This module has to be installed first, with
Next, you need to specify which functions you want to evaluate using the @profile decorator (you don’t need to import it in your file):
2 4 6 8 | def random_sort2(n): l.sort() random_sort2(2000000) |
Finally, you can obtain a line by line description of the random_sort2 function by typing:
where the -l flag indicates line-by-line and the -v flag indicates verbose output. With this method, we see that the array construction takes about 44% of the computation time, whereas the sort() method takes the remaining 56%.
You will also see that due to the time measurements, the script might take longer to execute.
6. Use the memory_profiler module
The memory_profiler module is used to measure memory usage in your code, on a line-by-line basis. However, it can make your code to run much more slower.
Install it with
Also, it is recommended to install the psutil package, so that the memory_profile runs faster:
In a similar way as the line_profiler, use the @profile decorator to mark which functions to track. Next, type:
yes, the previous script takes longer than the 1 or 2 seconds that took before. And if you didn’t install the psutil package, maybe you’re still waiting for the results!
Looking at the output, note that the memory usage is expressed in terms of MiB, which stand for mebibyte (1MiB = 1.05MB).
7. Using the guppy package
Finally, with this package you’ll be able to track how many objects of each type (str, tuple, dict, etc) are created at each stage in your code.
Python Slots Speed Play
Install it with
Next, add it in your code as:
2 4 6 8 10 12 14 | def random_sort3(n): print'Heap at the beginning of the functionn',hp.heap() l.sort() print'Heap at the end of the functionn',hp.heap() if__name__'__main__': |
And run your code with:
You’ll see something like the following output:
By placing the heap at different places in your code, you can study the object creation and deletion in the script flow.
Python Slots Speed Game
If you want to learn more about speeding your Python code, I recommend you the book High Performance Python: Practical Performant Programming for Humans, september 2014.
Hope it was useful! 🙂
Python Slots Speed Games
Don’t forget to share it with your friends!